Back to blog
Monday, November 18, 2024
How to create an AI agent that can fetch and analyze Yelp reviews
Posted by
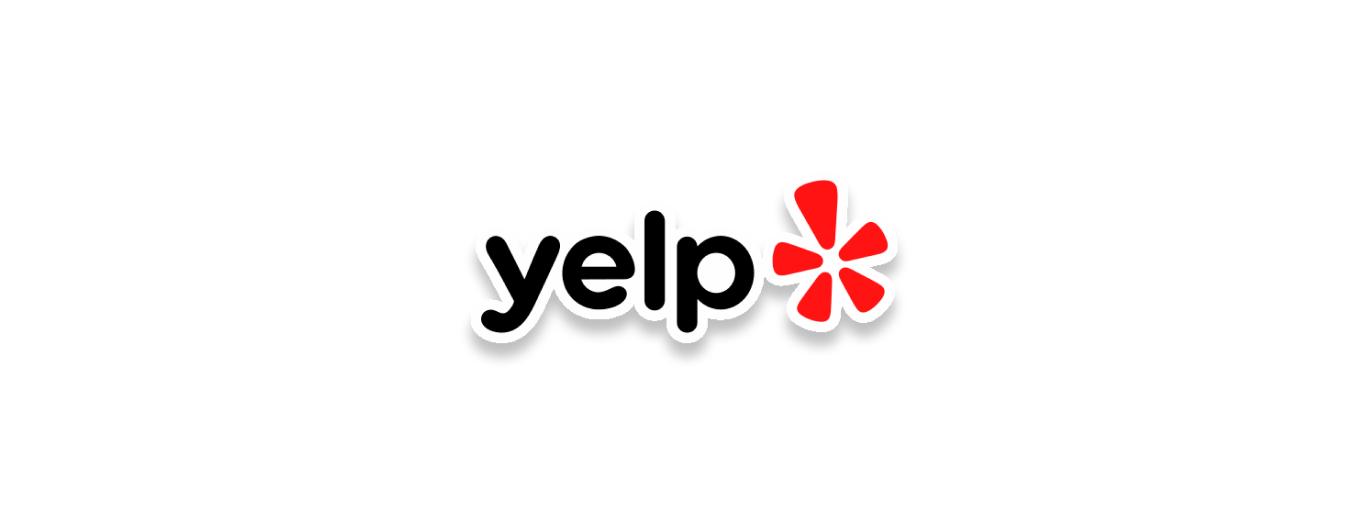
Getting Started
Install packages
pip install exfunc langgraph langchain langchain-openai
Configure API keys
You will need to provide API keys. You can get your Exfunc API key here and your OpenAI API key here.
Ensure both API keys are accessible in your local environment.
export EXFUNC_API_KEY=...
export OPENAI_API_KEY=...
Configure clients
from exfunc import Exfunc
from langchain_openai import ChatOpenAI
exfunc = Exfunc()
llm = ChatOpenAI(model="gpt-4o-mini")
Creating a ReAct Agent
We will create a ReAct agent using a prebuilt template by LangGraph. Given a user query, the agent runs in a loop:
- Decide if the query requires tool usage based on its requirements.
- If tool use is required:
- Identify and select the most appropriate tool for the task.
- Generate the necessary input for the tool and invoke it.
- Analyze the tool’s output to determine if additional tools are required.
- If further tools are needed, repeat step 2.
- If no tool use is needed or if the output sufficiently addresses the query, provide a response.
Define custom tools
We will add Exfunc's Yelp SearchBusinesses and GetBusinessReviews API as custom tools.
from langchain_core.tools import tool
@tool
def search_businesses(query: str, location: str) -> list:
"""Search businesses on Yelp."""
search_businesses_result = exfunc.yelp.search_businesses(request={
"query": query,
"location": location,
})
return search_businesses_result.businesses
@tool
def get_business_reviews(business_id: str) -> dict:
"""Get a list of reviews on Yelp for a given business ID."""
get_business_reviews_result = exfunc.yelp.get_business_reviews(request={
"business_id": business_id,
})
return get_business_reviews_result.reviews
tools = [search_businesses, get_business_reviews]
Create agent
from langgraph.prebuilt import create_react_agent
agent = create_react_agent(llm, tools)
Query agent
We will ask the AI agent to analyze reviews for Yin Du Wonton Noodle.
def print_stream(stream):
for s in stream:
message = s["messages"][-1]
if isinstance(message, tuple):
print(message)
else:
message.pretty_print()
query = "analyze reviews of yin du wonton noodle soup in SF"
inputs = {"messages": [("user", query)]}
print_stream(agent.stream(inputs, stream_mode="values"))
Example Response
================================ Human Message =================================
analyze reviews of yin du wonton noodle soup in SF
================================== Ai Message ==================================
Tool Calls:
search_businesses (call_l4...)
Call ID: call_l4...
Args:
query: Yin Du Wonton Noodle Soup
location: San Francisco
================================= Tool Message =================================
Name: search_businesses
**Redacted**
================================== Ai Message ==================================
Tool Calls:
get_business_reviews (call_XK...)
Call ID: call_XK...
Args:
business_id: TS...
================================= Tool Message =================================
Name: get_business_reviews
**Redacted**
================================== Ai Message ==================================
**Yin Du Wonton Noodle Soup** in San Francisco has garnered a mixture of reviews, reflecting both its authentic character and some areas of concern. Here’s an overview of the insights based on customer feedback:
### Overall Rating
- **Rating:** 3.7/5 based on 456 reviews
### Positive Aspects
1. **Authenticity:** Many customers appreciate the authentic feel of the restaurant, often commenting on its classic Chinatown vibe, including cash-only transactions and communal seating.
2. **Quality of Food:** The food, particularly the wonton and noodle soups, receive praise for being homey and comforting. Dishes like the Wonton Shrimp & Pork Soup and Stewed Beef Rice Noodle Soup are frequently mentioned for their rich flavors and hearty portions.
3. **Value for Money:** Several reviews highlight the value, noting that the prices are reasonable for the amount and quality of food provided, especially in a city like San Francisco.
4. **Quick Service:** Despite the sometimes chaotic atmosphere, many customers note that service is quick, with meals arriving promptly even during busy hours.
### Areas for Improvement
1. **Service Issues:** Some reviewers mention that the service can be disorganized, with staff being too busy to attend to customers promptly. This is a common theme, suggesting that while the food is good, the customer service experience may not be as high a priority.
2. **Ambience:** The restaurant's ambiance is described as simple and no-frills, which may not appeal to everyone. A few reviews indicate that cleanliness could be improved.
3. **Inconsistent Experiences:** While many reviews are positive, there are mentions of inconsistency in food quality, with some dishes being deemed average or lacking standout flavors.
### Notable Reviews
- **Redacted (4 stars):** Enjoyed the "home-cooked" flavor of the Wonton Shrimp & Pork Soup but noted the chaotic service.
- **Redacted (5 stars):** Described the egg noodles as "bouncing" and highlighted the deliciousness of the beef stew and other menu items.
- **Redacted (4 stars):** Loved the rich, flavorful broth of the stewed beef rice noodle soup, although noted that one wonton was missing filling.
- **Redacted (3 stars):** Found the food decent but nothing standout, emphasizing the cash-only policy and average cleanliness.
- **Redacted (4 stars):** Praised the beef brisket for its tenderness and depth of flavor but noted some issues with wonton fillings.
### Conclusion
Yin Du Wonton Noodle Soup is a beloved local spot known for its authentic flavors and quick service, despite some service-related complaints. It’s a great choice for those looking to enjoy hearty wonton noodles in a classic Chinatown setting, but diners should be prepared for a casual dining experience with potentially long wait times during peak hours.
Conclusion
You've now built an AI agent that can fetch and analyze Yelp reviews of a restaurant.
If you have any questions or need help, feel free to reach out to us at support@exfunc.com