Back to blog
Monday, December 2, 2024
Generate a TLDR of Google search results on Trump cabinet picks
Posted by
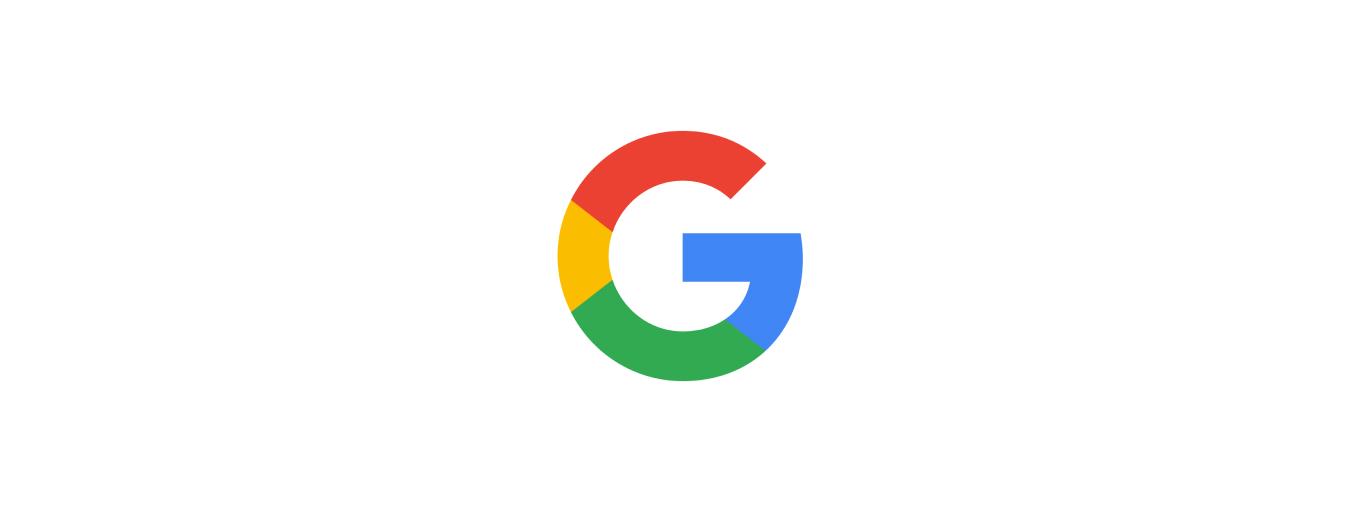
Getting Started
Install packages
pip install exfunc openai
Configure API keys
You will need to provide API keys. You can get your Exfunc API key here and your OpenAI API key here.
Ensure both API keys are accessible in your local environment.
import getpass
import os
if "OPENAI_API_KEY" not in os.environ:
os.environ["OPENAI_API_KEY"] = getpass.getpass("OpenAI API key:\n")
if "EXFUNC_API_KEY" not in os.environ:
os.environ["EXFUNC_API_KEY"] = getpass.getpass("Exfunc API key:\n")
Configure clients
from exfunc import Exfunc
from openai import OpenAI
exfunc = Exfunc()
openai = OpenAI()
Generating a TLDR of Google search results
Search web
search_web_response = exfunc.google.search_web(request={
"query": "trump cabinet picks",
"count": 10,
})
urls = [item.url for item in search_web_response.results]
Get articles in markdown
from concurrent.futures import ThreadPoolExecutor
def get_markdown(url):
max_len = 10000
try:
response = exfunc.navigator.scrape(request={"url": url})
return response.markdown[:max_len]
except:
return None
markdowns = []
with ThreadPoolExecutor(max_workers=min(10, len(urls))) as executor:
for markdown in executor.map(get_markdown, urls):
if markdown:
markdowns.append(markdown)
Generate TLDR
response = openai.chat.completions.create(
model="gpt-4o-mini",
messages=[
{"role": "system", "content": "TLDR in bullet points."},
{"role": "user", "content": "\n\n".join(markdowns)}
],
temperature=0
)
completion = response.choices[0].message.content.strip()
print(completion)
- **Trump's Cabinet Picks**: President-elect Donald Trump has announced several key appointments for his upcoming administration, focusing on loyalists and allies.
- **Key Appointments**:
- **Secretary of State**: Marco Rubio (Senator from Florida)
- **Defense Secretary**: Pete Hegseth (Fox News host and military veteran)
- **Attorney General**: Pam Bondi (former Florida Attorney General)
- **Health and Human Services Secretary**: Robert F. Kennedy Jr. (vaccine skeptic)
- **Treasury Secretary**: Scott Bessent (hedge fund manager)
- **Interior Secretary**: Doug Burgum (Governor of North Dakota)
- **Agriculture Secretary**: Brooke Rollins (former White House aide)
- **Commerce Secretary**: Howard Lutnick (financial services executive)
- **Labor Secretary**: Lori Chavez-DeRemer (former Congresswoman)
- **EPA Administrator**: Lee Zeldin (former Congressman)
- **U.N. Ambassador**: Elise Stefanik (Congresswoman)
- **CIA Director**: John Ratcliffe (former Congressman)
- **Director of National Intelligence**: Tulsi Gabbard (former Congresswoman)
- **FBI Director**: Kash Patel (former Trump aide)
- **Ambassador to France**: Charles Kushner (real estate developer)
- **Ambassador to the UK**: Warren Stephens (investment banker)
- **Controversial Picks**: Some nominees, like Robert F. Kennedy Jr. and Pete Hegseth, have faced scrutiny due to their controversial views and past allegations.
- **Withdrawal**: Former Rep. Matt Gaetz withdrew from consideration for Attorney General amid controversy.
- **Senate Confirmation**: Most of these appointments will require Senate confirmation, which may be facilitated by a Republican-controlled Senate.
- **Focus on Loyalty**: Trump's selections reflect a strategy of surrounding himself with individuals who have demonstrated loyalty to him and his policies.
Conclusion
You have now generated a TLDR of Google search results on Trump cabinet picks
If you have any questions or need help, feel free to reach out to us at support@exfunc.com